Literate Programming and Donald Knuth
I was first introduced to this concept by Distrotube (Derek Taylor's) "literate config" files. At the time I was not using emacs
and thus all the code I was writing was sparingly commented.
Since then, I have entered a world of Machine Learning and Deep Learning, where suddenly in 4 lines, I can sit atop my high-horse and perform sentiment analysis with tensorflow and keras!
from transformers import pipeline
classifier = pipeline('sentiment-analysis')
prediction = classifier("Donald Knuth was the greatest computer scientist.")[0]
print(prediction)
Device set to use mps:0 {'label': 'POSITIVE', 'score': 0.9997720122337341}
In such an age of abstraction complexity, it becomes paramount to distill what is happening at the last few \((n-k)\) layers.
Abstraction Inversion
Literate programming is a methodology introduced by Donald E. Knuth in the early 1980s that introduced a paradigm-shift: it allowed developers to reconsider code
as being a means to the end as opposed to being the end itself.
What I mean by this, is that suddenly code didn't become about talking to the computer, it became about talking to other developers. Including, importantly talking to your future-self!
Tangibly, this meant inverting the structure of a program and writing prose imbued with code, as opposed to code imbued with prose, or as known to you a-priori—"comments".
This approach brings documentation, explanation, design justifications to the forefront and enhances maintainability, teaching value, and clarity.
Origins
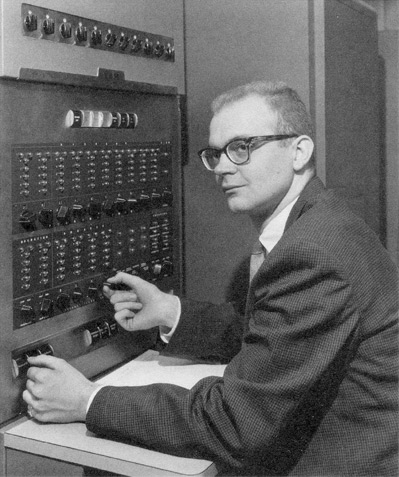
Donald Knuth, the renowned computer scientist best known for his work on the monumental The Art of Computer Programming, introduced the concept of literate programming in 1984 through his system called WEB. According to Knuth, traditional programming methods often inhibit the readability and maintainability of the source code. In WEB (which originally targeted Pascal, then later adapted to C and other languages), a single file contained both explanatory text and code sections. A separate preprocessor would then produce two outputs:
- Tangled output – This contained the machine-readable source code that could be compiled or interpreted.
- Woven output – This was a richly formatted document that provided a human-readable narrative including the code.
Knuth's philosophy centered on viewing programs as works of literature, composed primarily for human beings rather than just compilers or interpreters. He believed this perspective better captures the creative process of programming and encourages developers to articulate why certain design choices were made. The result is a more maintainable, accessible, and instructive codebase within which the entry activation threshold is lowered and thus the learning curve is accelerated for new developers. A humbling but effective practice!
Porcelain
In the days of assembly language or whenever the ancient Prof. Knuth employed Literate Programming, there were not such finely attuned and robust tools such as Emacs and Org-mode to glide through with. Nowadays we have Babel with syntax highlighting and embedded code-blocks which can be tangled into language-specific files, i.e. .py
or .c
, etc. Furthermore, org supports active documents whithin which code can be executed and results captured directly within the same file! Additionally, many languages may be included within the same file..
The power of Emacs is itself extreme; often it is likened to an entire operating system wherein you may check your mail, browse internet, write code and even change the very structure of the land you are standing on, as you stand upon it. All in all, it is a very lucrative and liquid ocean.
The synergy of Emacs and Org-mode forms a potent combination for literate programming.
Lastly, there is a fantastic screencast by Howard Abrams on the topic which summarises and extends my notes here on the topic: