Shell/s
There are many shells, all of them do the same thing — they allow you to manipulate your filesystem.
I have experience with all of the major shells excluding Powershell1. ChatGPT has written this thousand word explainer, and I have made the people orange, the places purple and the softwares blue :).
The Bourne shell (sh)
Origins
The Stephen Bourne Shell first appeared in Version 7 Unix (1979) at Bell Labs. It replaced the earlier Thompson shell and became the standard shell for Unix systems in the late 1970s and 1980s. Its design introduced structured programming features (variables, control flow) to improve on the limitations of older shells.
Uses
By default, /bin/sh
was the login shell on historical Unix systems. Today, “sh” usually refers to a POSIX-compliant shell, widely used for portability. For instance, many distributions link /bin/sh
to dash. The Bourne shell (sh) remains crucial for init scripts, install scripts, and other tasks demanding universal compatibility.
Advantages
- Universal Compatibility: A POSIX
sh
script runs on nearly any Unix-like system. - Minimal Resource Usage: Implementations like dash are lightweight and start quickly.
- Simplicity: Fewer constructs facilitate easier auditing.
- Foundation for Other Shells: Mastery of The Bourne shell syntax translates to Bash, Zsh, etc.
Disadvantages
- Limited Interactivity: Traditional
sh
lacks history or tab completion. - Few Advanced Features: No arrays or rich expansions.
- Implementation Variance:
/bin/sh
might be different shells on different systems. - Small Ecosystem: Relies on external tools for most functionality.
Capabilities
sh
handles basic control flow (loops, if statements), pipes, redirection, variables, and command substitution. Scripts often leverage other Unix utilities for string manipulation and arithmetic.
#!/bin/sh
echo "Script name: $0"
for arg in "$@"; do
echo "Arg: $arg"
done
Limitations
- No array type.
- Minimal expansions; integer math often requires external tools (
expr
). - Weak error handling.
- Poor for interactive use (no line editing by default).
Bash (Bourne Again Shell)
Origins
Brian Fox created Bash in 1989 for the GNU Project, later maintained by Chet Ramey. It was designed to be a free replacement for The Bourne shell with features from C shell and KornShell. It’s the default interactive shell on many Linux distros and was the default on macOS until recent updates.
Uses
Used both interactively and for scripting, Bash is ubiquitous in the Linux/Unix world. System boot scripts and user dotfiles (~/.bashrc
) rely on it. It’s also the standard shell in many Docker images and widely adopted for CI/CD pipelines.
Advantages
- Widespread Availability: Preinstalled on most Linux systems.
- Feature-Rich Scripting: Arrays (indexed/associative), brace expansion, enhanced parameter substitution.
- Interactive Tools: Command history, line editing, programmable tab completion.
- POSIX Compatibility (with Extensions): Smoothly runs many
sh
scripts.
Disadvantages
- Configuration Required: By default lacks modern niceties like autosuggestions or highlighting.
- Inherited Syntax Quirks: Complex expansions, quoting, and subtle pitfalls.
- Performance in Large Scripts: Spawning subshells for pipelines can slow complex tasks.
- Version Discrepancies: Some systems ship older releases (e.g., macOS 3.2 vs. 5.x on Linux).
Capabilities
Bash extends sh
with features like arrays, [[...]]
tests, arithmetic expansion, and process substitution. Ideal for moderate automation and daily command-line use.
#!/usr/bin/env bash
fruits=(apple banana cherry)
for fruit in "${fruits[@]}"; do
echo "$fruit"
done
Limitations
- Depends on presence of Bash (not always default on minimal systems).
- Script portability can break if you rely on non-POSIX features.
- No built-in floating-point or complex data structures.
- Error handling limited to exit codes and traps.
Z shell (Zsh)
Origins
Paul Falstad developed Zsh around 1990, naming it after a Yale professor’s login ID. Zsh merged ideas from KornShell, C shell, and sh
, eventually evolving into a highly customizable, feature-rich shell. Zsh became the default on macOS starting with Catalina and is also default on Kali Linux.
Uses
Advantages
- Feature Superset: Extended globbing, floating-point math, advanced array handling.
- Programmable Completions: Completion system can correct spelling and handle command-specific completions.
- Customization & Theming: Powerful prompt expansions and community plugins via frameworks.
- Good Bash Compatibility: Many Bash scripts work unmodified under Zsh.
Disadvantages
- Complex Setup: Defaults are plain; advanced features typically need user configuration or plugins.
- Minor Bash Differences: Some corner cases or built-ins differ slightly from Bash.
- Availability: Not always installed on minimal systems.
- Potential Overhead: Numerous features, plus plugins, can slow startup relative to simpler shells.
Capabilities
Zsh supports advanced glob qualifiers, theming, spelling corrections, and can emulate POSIX or sh
if desired. Scripting can employ extended features or remain portable.
# Inside ~/.zshrc:
autoload -U compinit && compinit
PROMPT='%n@%m:%~%# '
setopt autocd
Limitations
- Requires manual enabling of extras like syntax highlighting, autosuggestions.
- Large configuration surface (zshrc, plugins, etc.).
- Slight performance overhead vs. minimal shells.
- Not universal on all systems.
Fish (Friendly Interactive Shell)
Origins
Axel Liljencrantz first released Fish in 2005, aiming for a modern, user-friendly shell free from historical POSIX constraints. Fish is open-source (GPL) and developed by a dedicated community, embracing simplicity and discoverability over strict backward compatibility.
Uses
Fish is mainly an interactive shell that provides autosuggestions, syntax highlighting, and convenient defaults. It’s not intended for system scripting but can be used if all target environments have Fish installed. Developers enjoy its user-centric features for daily command-line tasks.
Advantages
- User-Friendly Defaults: Syntax highlighting and inline autosuggestions out of the box.
- Consistent Syntax: Avoids many Bash quirks (e.g., no tricky variable or function definitions).
- Rich Tab Completion: Parses man pages for context-aware completions with descriptions.
- Modern Interaction: Web-based configuration, universal variables, and real-time error checking.
Disadvantages
- Non-POSIX: Scripts are Fish-specific; cannot run standard
sh
or Bash scripts verbatim. - Smaller Ecosystem: Fewer community frameworks than Zsh or Bash.
- Requires Installation: Rarely preinstalled; must be installed manually.
- Different Scripting Style: Seasoned shell users must relearn certain syntax.
Capabilities
Fish excels interactively, providing colored prompts, advanced tab completion, and no subshell forks in pipelines. Configuration typically resides in ~/.config/fish/config.fish
. Its scripting uses straightforward commands: set
for variables, function ... end
for functions.
# Fish example:
set greeting "Hello from Fish!"
echo $greeting
function say_hi
echo "This is a fish function"
end
say_hi
Limitations
- No POSIX compatibility mode.
- Missing certain shell features (like traps or $LINENO).
- Possibly slower startup due to extensive completion logic.
- Not a default on most systems.
Eshell (Emacs Shell)
Origins
John Wiegley created Eshell in the late 1990s. Entirely written in Emacs Lisp, it’s bundled with GNU Emacs. Eshell aims to provide a shell-like environment within Emacs, working consistently across Windows, macOS, and Linux.
Uses
Eshell is used from inside Emacs, letting developers run commands, manipulate files, and even work with remote directories via Tramp. It’s purely an interactive tool rather than a standalone system shell; you cannot ssh
directly into Eshell.
Advantages
- Deep Emacs Integration: Can call Emacs functions inline, like
(message \"Hello\")
or(find-file)
. - Cross-Platform Consistency: Implements many Unix utilities in Lisp, bridging gaps on systems lacking them.
- Extensibility: Functions can be overridden or defined in Emacs Lisp.
- Multiple Sessions: Each
Eshell
buffer is independent, and you can easily create more.
Disadvantages
- Emacs Dependent: Useless outside GNU Emacs, can’t serve as
/bin/sh
. - Performance Constraints: Large outputs or directories may slow the editor.
- Not Fully POSIX-Compliant: Some expansions or advanced shell features differ from standard shells.
- Terminal Emulation Limits: Curses-like apps often need a separate
ansi-term
buffer.
Capabilities
Eshell runs external commands or built-in Lisp versions of commands like ls
, grep
, etc. It supports pipes, redirection, and mixing Emacs Lisp expressions inline. Output appears in an Emacs buffer, making it straightforward to copy, edit, or search results. Remote directories work seamlessly with Tramp
.
;; Example Eshell usage:
echo "Home: $HOME"
(message "Hello from Emacs Lisp!")
find-file ~/notes.org
Limitations
- Not suitable for typical system scripting or login shells.
- No standard job control (Ctrl-Z, fg/bg) akin to normal terminals.
- Different syntax in places (partially Lisp-based).
- Entirely reliant on Emacs being open.
Conclusion
Finally, I would like to add that each instance of the shell
is atomic (regardless of which particular flavour you choose).
As such, if you are not using a terminal multiplexer such as tmux, then you are an idiot.
Here is a preview of what my \(0^\text{th}\) session windows looked like back in 2022:
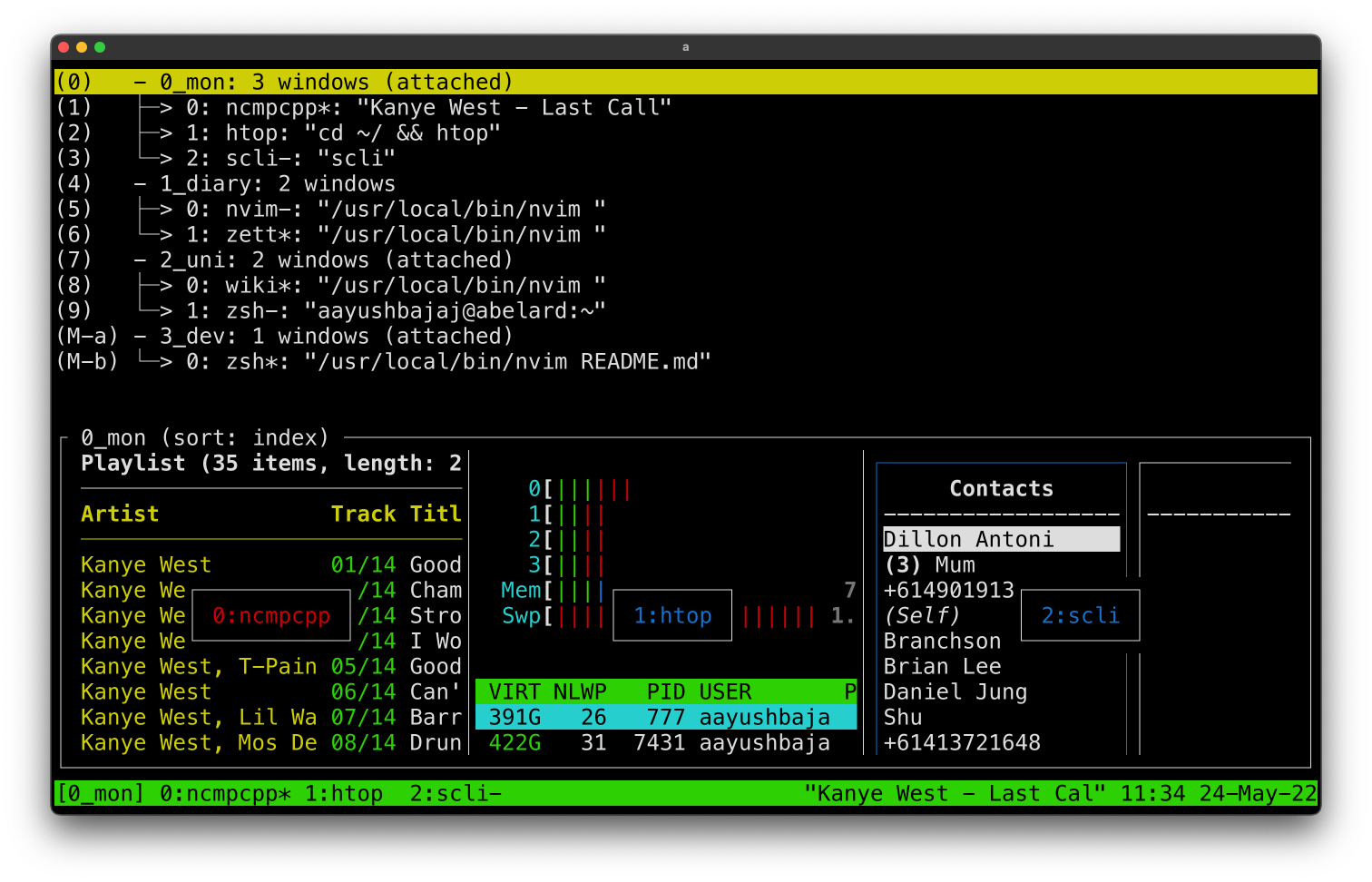
Footnotes
as of 07/03/25