History
Abstract
Fashion-MNIST is a modern drop-in replacement for MNIST. Released by Zalando Research in 2017, it packs 70 000 tiny grayscale images of apparel—sneakers, shirts, coats—into a lightweight benchmark. Its familiar format keeps setup trivial, while richer visuals pose a tougher challenge.
Origins
Zalando’s quality-control cameras captured millions of 96 × 96 product shots. Han Xiao et al. down-sampled these to 28 × 28, grouped them into ten balanced classes, and open-sourced the result. The idea: upgrade MNIST difficulty without touching loaders or evaluation scripts.
This page includes ⊕ my Chapter notes for the book by Michael Nielsen.
We have seen what can be learned by the perceptron algorithm — namely, linear decision boundaries for binary classification problems.
It may also be of interest to know that the perceptron algorithm can also be used for regression with the simple modification of not applying an activation function (i.e. the sigmoid). I refer the interested reader to open another tab.
We begin with the punchline:
XOR
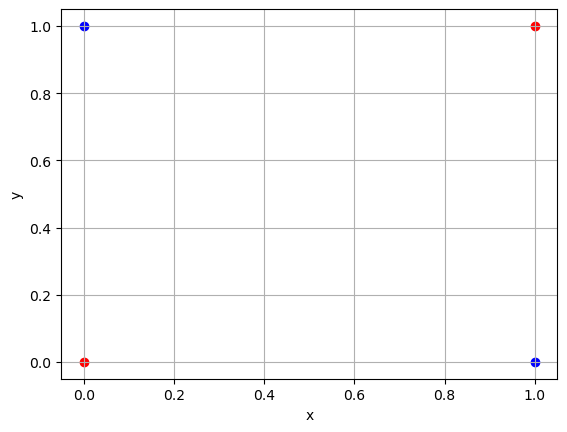
Now clearly, taking a ruler, your finger or positioning any straight-lined object on the above figure will not enable you to separate the blue (true) from the red (false) circles. This was also one of Marvin Minsky's arguments against further development of the Perceptron in 1963. However, with the benefit of hindsight, we shall not retire so quickly, instead we add another layer of the neurons: